Tutorial Intro
Let's discover react-native-echarts-pro in less than 5 minutes.
Getting Started
npm install react-native-echarts-pro --save
or
yarn add react-native-echarts-pro
What you'll need
react-native
- version 0.59 or above.
react-native-webview
- Below
1.9.0
must be greater than or equal to6.9.0
- Above
1.9.0
must be greater than or equal to11.26.0
Usage
Base
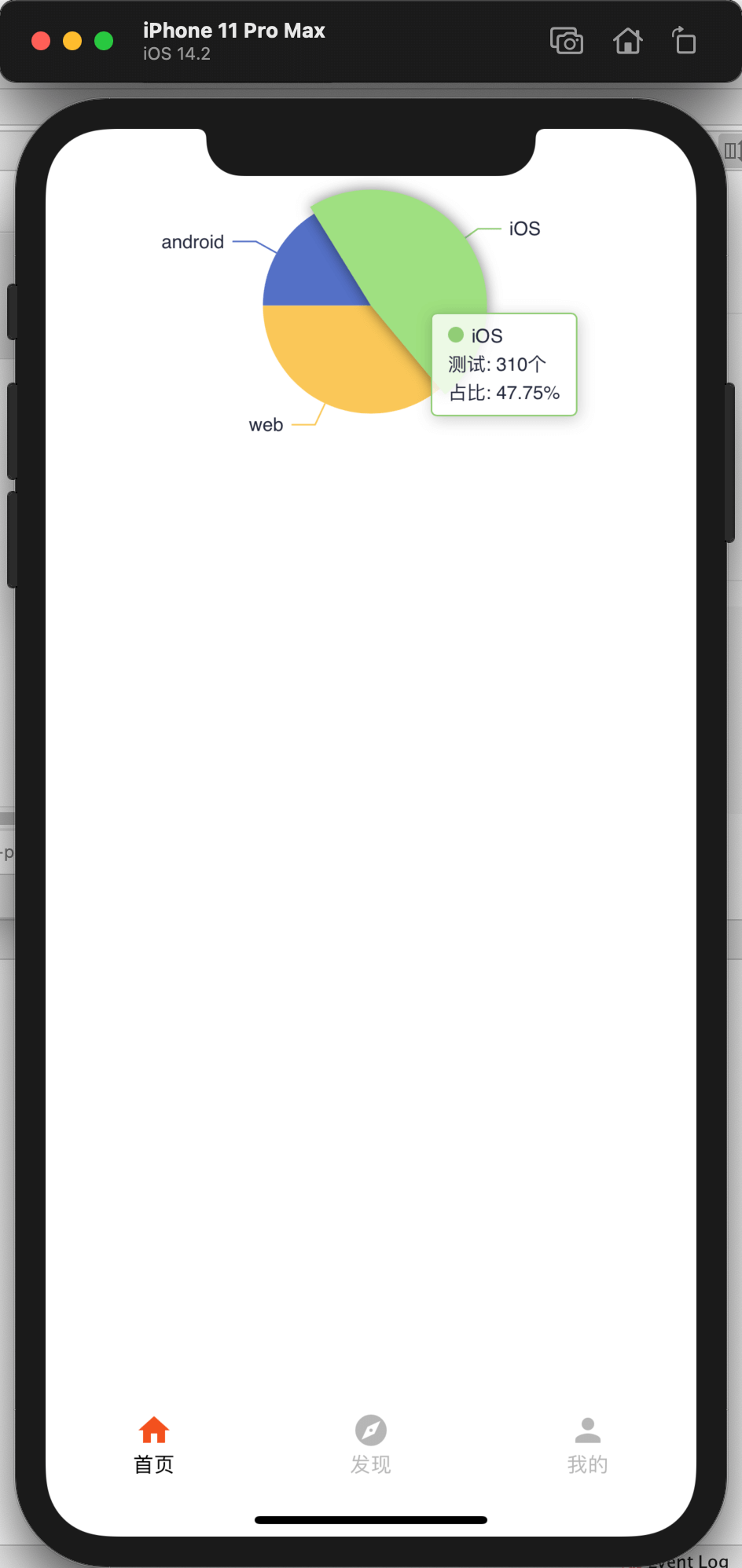
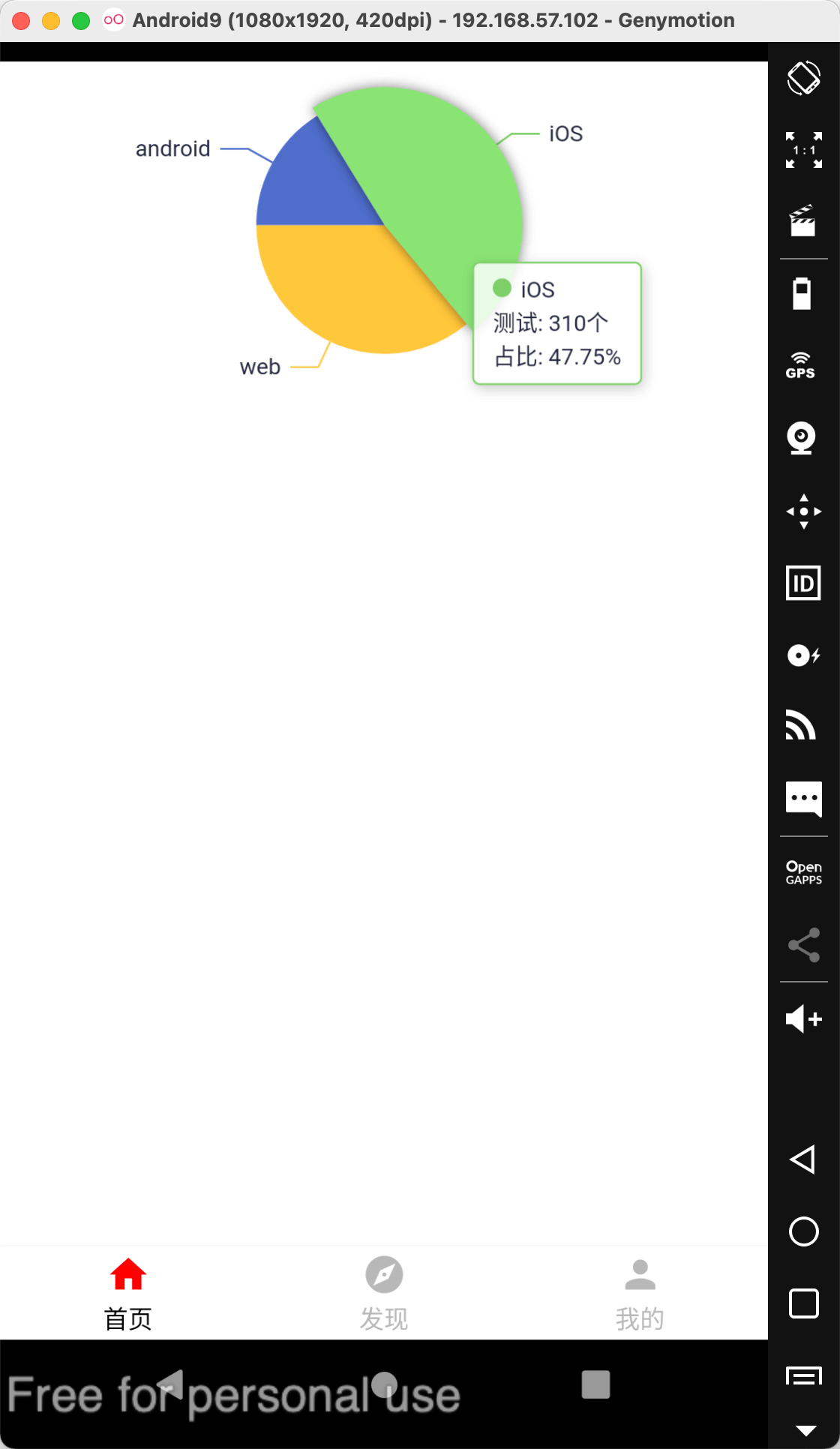
import React from "react";
import { View } from "react-native";
import RNEChartsPro from "react-native-echarts-pro";
export default function RNEPDemo() {
const pieOption = {
series: [
{
name: "Source",
type: "pie",
legendHoverLink: true,
hoverAnimation: true,
avoidLabelOverlap: true,
startAngle: 180,
radius: "55%",
center: ["50%", "35%"],
data: [
{ value: 105.2, name: "android" },
{ value: 310, name: "iOS" },
{ value: 234, name: "web" },
],
label: {
normal: {
show: true,
textStyle: {
fontSize: 12,
color: "#23273C",
},
},
},
},
],
};
return (
<View style={{ height: 300, paddingTop: 25 }}>
<RNEChartsPro height={250} option={pieOption} />
</View>
);
}
Map
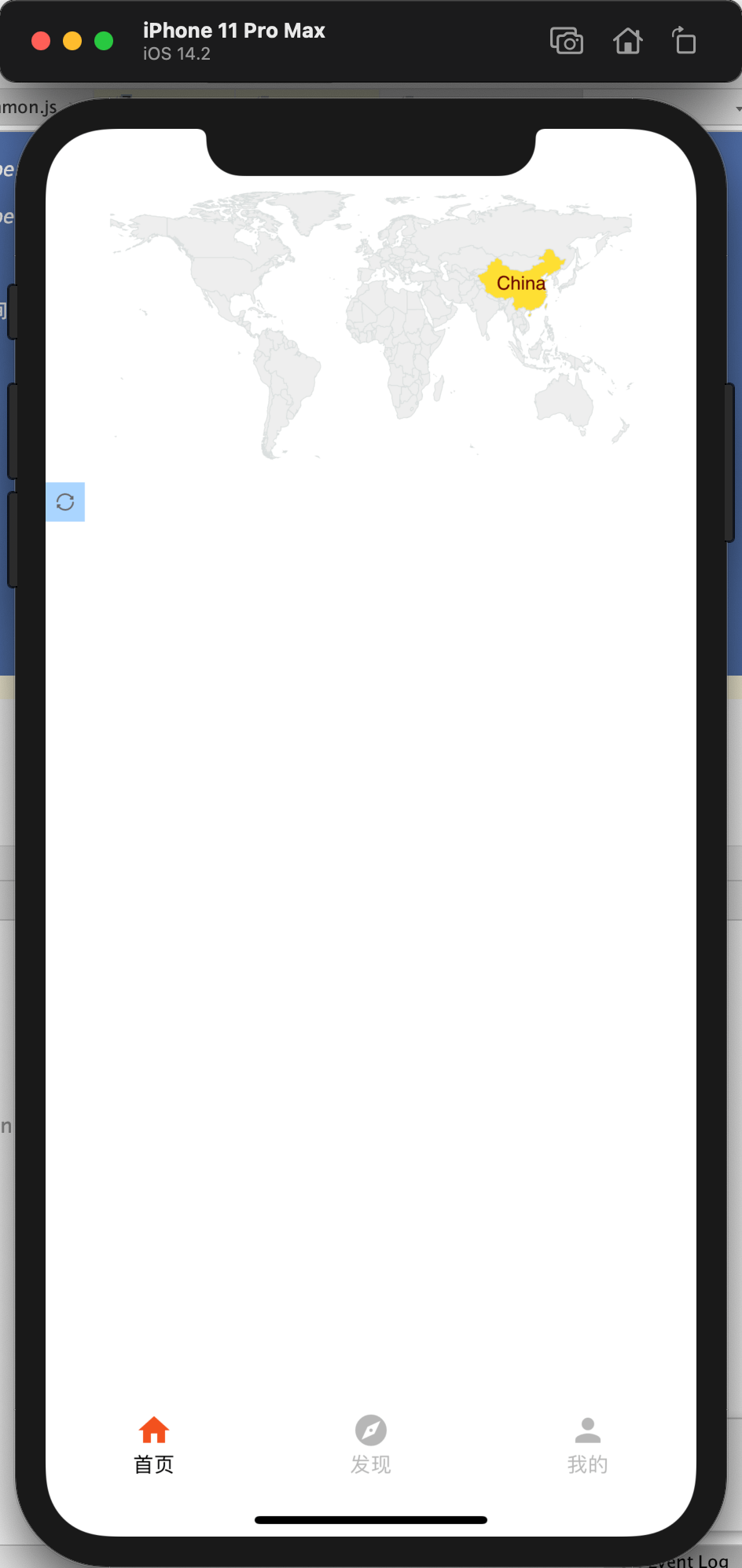
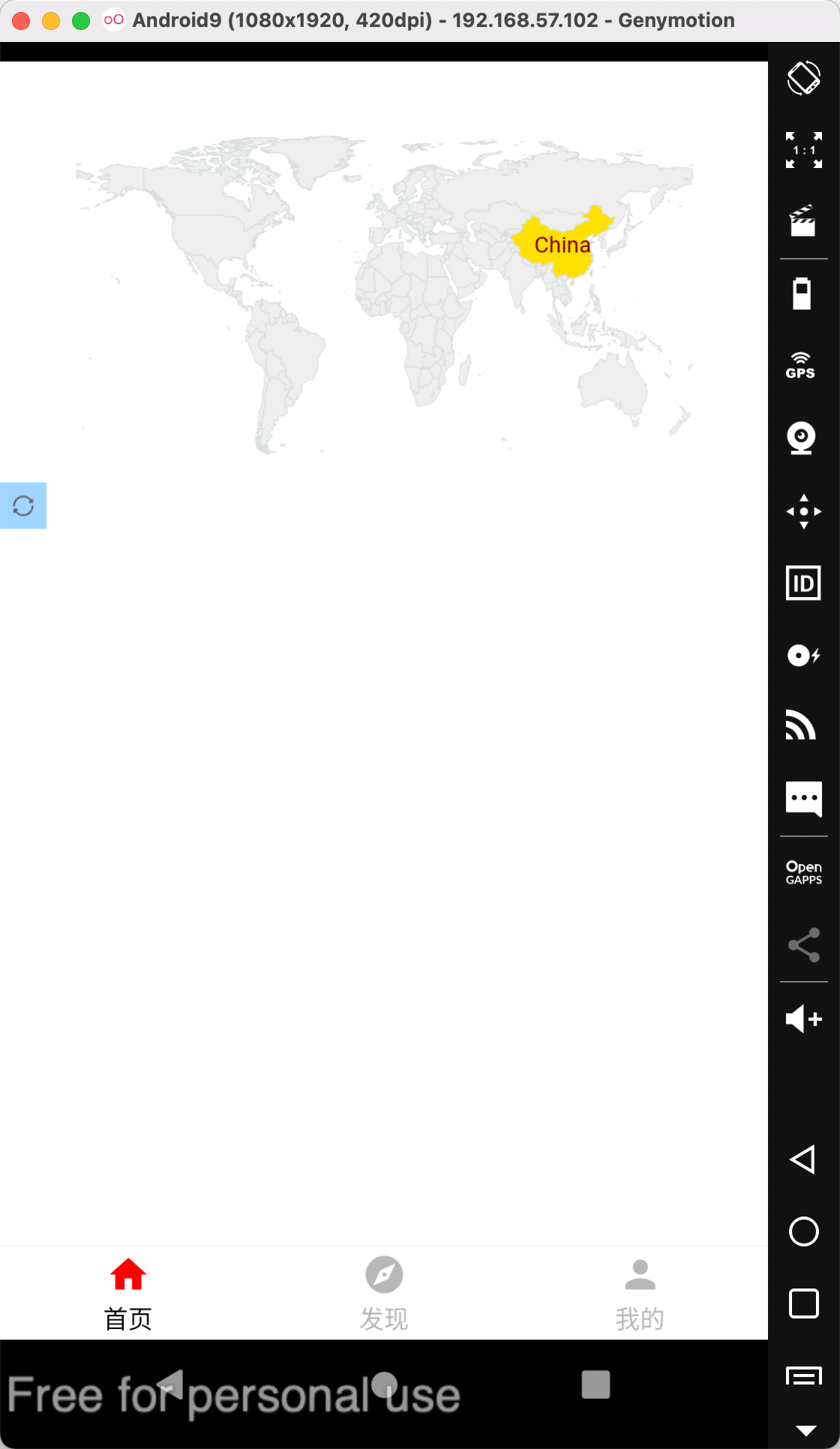
import React from "react";
import { View } from "react-native";
import RNEChartsPro from "react-native-echarts-pro";
export default function Demo() {
const mapData = {
visualMap: {
show: true ,
left: "right",
top: "center",
min: 1,
max: 3,
calculable: true,
},
geo: [
{
type: "map",
map: "world",
mapType: "world",
selectedMode: "single",
itemStyle: {
normal: {
areaStyle: { color: "#B1D0EC" },
color: "#eeeeee",
borderColor: "#dadfde",
},
emphasis: {
//mouse hover style
label: {
show: true,
textStyle: {
color: "#000000",
},
},
},
},
roam: true,
},
],
series: {
type: "effectScatter",
coordinateSystem: "geo",
geoIndex: 0,
itemStyle: {
color: "red",
},
data: [[116.4551, 40.2539, 10]],
},
toolbox: {
show: true,
orient: "horizontal",
x: "left",
y: "bottom",
backgroundColor: "#1e90ff60",
itemGap: 10,
itemSize: 10,
color: "#ffffff",
showTitle: false,
feature: {
restore: {
show: true,
title: "Reset",
},
},
},
};
return (
<View style={{ height: 300 }}>
<RNEChartsPro height={250} option={mapData} />
</View>
);
}